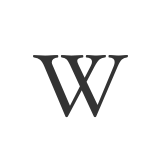
复杂度
强制程序中允许的最大循环复杂度
循环复杂度衡量程序源代码中线性独立路径的数量。此规则允许设置循环复杂度阈值。
function a(x) {
if (true) {
return x; // 1st path
} else if (false) {
return x+1; // 2nd path
} else {
return 4; // 3rd path
}
}
规则详情
此规则旨在通过限制程序中允许的循环复杂度来降低代码复杂度。因此,当循环复杂度超过配置的阈值(默认为 `20`)时,它会发出警告。
最大值为 `2` 的 **错误** 代码示例
在 Playground 中打开
/*eslint complexity: ["error", 2]*/
最大值为 `2` 的 **正确** 代码示例
在 Playground 中打开
/*eslint complexity: ["error", 2]*/
function a(x) {
if (true) {
return x;
} else {
return 4;
}
}
function b() {
foo ||= 1;
}
类字段初始化器和类静态块是隐式函数。因此,它们的复杂度是为每个初始化器和每个静态块单独计算的,并且它不会影响封闭代码的复杂度。
最大值为 `2` 的其他 **错误** 代码示例
在 Playground 中打开
/*eslint complexity: ["error", 2]*/
class C {
x = ; // this initializer has complexity = 3
}
class D { // this static block has complexity = 3
}
最大值为 `2` 的其他 **正确** 代码示例
在 Playground 中打开
/*eslint complexity: ["error", 2]*/
function foo() { // this function has complexity = 1
class C {
x = a + b; // this initializer has complexity = 1
y = c || d; // this initializer has complexity = 2
z = e && f; // this initializer has complexity = 2
static p = g || h; // this initializer has complexity = 2
static q = i ? j : k; // this initializer has complexity = 2
static { // this static block has complexity = 2
if (foo) {
baz = bar;
}
}
static { // this static block has complexity = 2
qux = baz || quux;
}
}
}
选项
此规则有一个数字或对象选项
-
`"max"`(默认值:`20`)强制执行最大复杂度
-
`"variant": "classic" | "modified"`(默认值:`"classic"`)要使用的循环复杂度变体
最大值
使用 `max` 属性自定义阈值。
"complexity": ["error", { "max": 2 }]
**已弃用:** 对象属性 `maximum` 已弃用。请使用属性 `max` 代替。
或使用简写语法
"complexity": ["error", 2]
变体
要使用的循环复杂度变体
- `"classic"`(默认)- 经典 McCabe 循环复杂度
- `"modified"` - 修改后的循环复杂度
_修改后的循环复杂度_ 与经典循环复杂度相同,但每个 `switch` 语句仅将复杂度值增加 `1`,无论它包含多少个 `case` 语句。
使用 `{ "max": 3, "variant": "modified" }` 选项的此规则的 **正确** 代码示例
在 Playground 中打开
/*eslint complexity: ["error", {"max": 3, "variant": "modified"}]*/
function a(x) { // initial modified complexity is 1
switch (x) { // switch statement increases modified complexity by 1
case 1:
1;
break;
case 2:
2;
break;
case 3:
if (x === 'foo') { // if block increases modified complexity by 1
3;
}
break;
default:
4;
}
}
上述函数的经典循环复杂度为 `5`,但修改后的循环复杂度仅为 `3`。
何时不使用
如果您无法为您的代码确定合适的复杂度限制,那么最好禁用此规则。
相关规则
版本
此规则在 ESLint v0.0.9 中引入。
进一步阅读
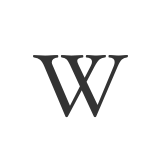
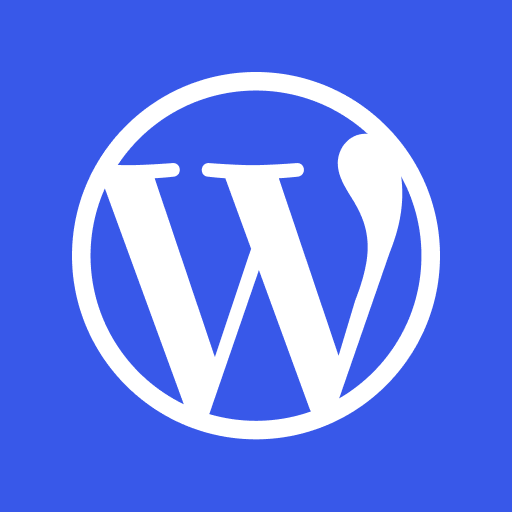
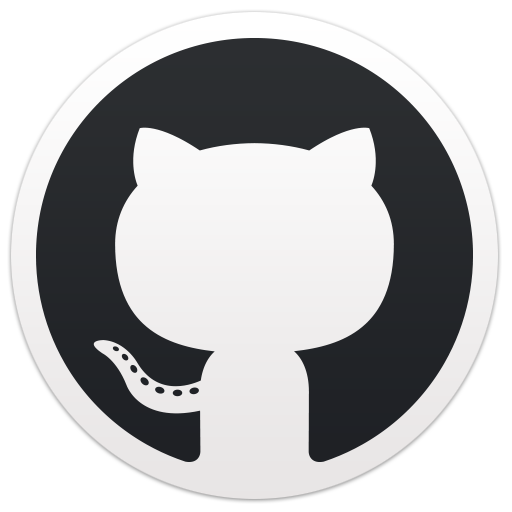